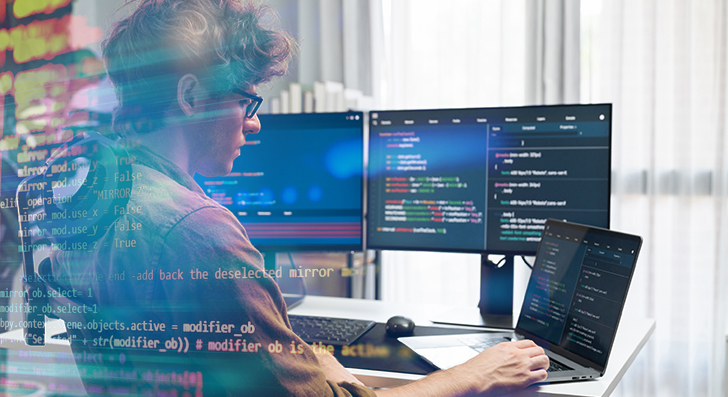
Scalability implies your software can take care of progress—much more users, additional knowledge, and a lot more site visitors—without breaking. Being a developer, developing with scalability in your mind saves time and stress later on. Right here’s a transparent and useful guide that will help you start by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's strategy from the start. Numerous apps fail if they develop rapid simply because the first style can’t handle the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Commence by coming up with your architecture to get adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting The full procedure.
Also, consider your databases from working day one. Will it require to deal with 1,000,000 people or simply just a hundred? Choose the correct style—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential stage is to stop hardcoding assumptions. Don’t generate code that only is effective under current conditions. Consider what would occur Should your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use design patterns that aid scaling, like information queues or party-pushed programs. These support your application cope with additional requests devoid of receiving overloaded.
If you Construct with scalability in mind, you're not just making ready for achievement—you are cutting down long run head aches. A nicely-planned procedure is simpler to keep up, adapt, and grow. It’s improved to arrange early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is really a key Component of making scalable apps. Not all databases are constructed the identical, and using the Erroneous one can gradual you down as well as result in failures as your application grows.
Start off by comprehending your details. Could it be hugely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective match. These are definitely sturdy with relationships, transactions, and regularity. They also support scaling tactics like study replicas, indexing, and partitioning to manage much more website traffic and information.
In the event your information is a lot more flexible—like consumer activity logs, product or service catalogs, or documents—look at a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, look at your study and publish styles. Are you currently executing lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a large write load? Explore databases which will tackle higher publish throughput, or maybe occasion-based mostly facts storage units like Apache Kafka (for temporary information streams).
It’s also good to think ahead. You may not want Innovative scaling capabilities now, but deciding on a databases that supports them means you won’t need to switch later on.
Use indexing to hurry up queries. Keep away from unwanted joins. Normalize or denormalize your details based upon your obtain styles. And normally monitor databases performance when you develop.
In brief, the correct database depends upon your app’s composition, velocity desires, And exactly how you be expecting it to increase. Just take time to select correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Stay clear of repeating logic and take away something unnecessary. Don’t pick the most sophisticated solution if a straightforward a single works. Keep the features shorter, centered, and simple to test. Use profiling instruments to discover bottlenecks—sites the place your code requires much too extended to operate or employs an excessive amount of memory.
Future, check out your database queries. These generally slow points down greater than the code alone. Make certain Each individual query only asks for the info you actually will need. Steer clear of Pick out *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent doing too many joins, In particular across huge tables.
For those who discover the exact same info staying asked for repeatedly, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app far more economical.
Make sure to test with big datasets. Code and queries that perform high-quality with a hundred documents may well crash whenever they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code tight, your queries lean, and use caching when wanted. These ways help your software continue to be sleek and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it has to deal with much more customers and even more site visitors. If almost everything goes by way of a single server, it is going to swiftly become a bottleneck. That’s exactly where load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing every one of the perform, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing details quickly so it may be reused rapidly. When buyers request exactly the same information and facts once again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it from your cache.
There's two widespread types of caching:
one. Server-side caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are easy but powerful equipment. Alongside one another, they help your app cope with more consumers, keep speedy, and Recuperate from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable programs, you need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors improves, you read more can add much more resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on building your application in place of taking care of infrastructure.
Containers are One more essential Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be effortless to move your application involving environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single portion of one's application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You could update or scale elements independently, which is perfect for overall performance and dependability.
In brief, working with cloud and container resources usually means you'll be able to scale speedy, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop with no limits, start off applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Keep an eye on All the things
In case you don’t observe your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is performing, spot troubles early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control just how long it will require for people to load internet pages, how frequently glitches transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified promptly. This will help you resolve concerns quick, often before buyers even detect.
Monitoring can also be useful after you make improvements. In case you deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper instruments in place, you continue to be in control.
To put it briefly, monitoring helps you maintain your app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it really works effectively, even stressed.
Last Views
Scalability isn’t just for major businesses. Even smaller apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you are able to Make apps that expand effortlessly with out breaking stressed. Begin modest, think huge, and Make smart.